ปรากฎว่าการใส่ * ในช่อง text ที่ใช้ search กลายเป็นการ enable feature searchwiki ไป
ดูตัวอย่าง
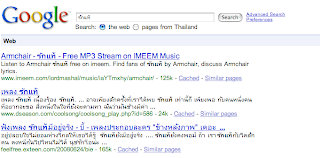
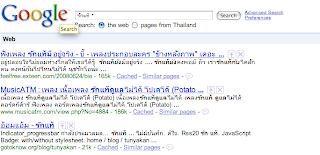
Javaเร็วส์, Javascript, Erlang, Python, Ruby, Clojure, Groovy, เลี้ยงลูก, วาดภาพ
(ns test
(:import (java.util.regex Pattern)))
(defstruct pos :x :y)
(defn explode
"return a pos/type seq for each char in the line"
[y line]
(map (fn [x y type] (vector (struct pos x y) type))
(iterate inc 0) (repeat y) line))
(defn line-explode
"call explode on each line with the appropriate y"
[lines]
(let [linesplit (. Pattern compile "\n" (. Pattern MULTILINE))] ; must be a better way?
(reduce into
(map explode
(iterate inc 0)
(. linesplit split lines)))))
(ns test
(:import (java.util.regex Pattern)))
(defn function-name description params body)
เข่น
(defn plus-9 "plus with 9" [x] (+ x 9))
(defn line-explode
"call explode on each line with the appropriate y"
[lines]
(let bindings body)
เช่น
(let [x 9, y 10] (+ x y))
run แล้วได้ผลลัพท์เป็น 19
ใน bindings ของ let นั้น, variable ที่ถูก bind ก่อน สามารถถูกอ้างถึงโดย variable ที่ bind ทีหลังได้
(let [x 9, y (+ x 7)] (+ x y))
run แล้วได้ผลลัพท์เป็น 25
(let [linesplit (. Pattern compile "\n" (. Pattern MULTILINE))] ; must be a better way?
Pattern linesplit = Pattern.compie("\n", Pattern.MULTILINE);
(reduce into
(map explode
(iterate inc 0)
(. linesplit split lines)))
(. linesplit split lines)
(iterate inc 0)
(map + '(1 2 3) '(3 4 5))
=> (4 6 8)
(map + '(1 2 3) '(3 4 5 6 7 8))
=> (4 6 8)
explode
ดังนั้นเราต้องตามไปดูว่ามันทำอะไร
(defn explode
"return a pos/type seq for each char in the line"
[y line]
(map (fn [x y type] (vector (struct pos x y) type))
(iterate inc 0) (repeat y) line))
fn
ในบรรทัดที่ 4 โดยมันคือการ define function อีกแบบหนึ่ง ซึ่งมีคุณสมบัติแบบ closure
test> (def foo
(let [y 10]
(fn [x] (+ x y))))
#=(var test/foo)
test> (foo 7)
17
ลองเปลี่ยน y ให้เป็น 12 ณ ขณะที่ run
test> (let [y 12] (foo 7))
17
repeat
นั่นเป็น infinite list อีกตัวหนึ่ง(repeat 7)
=> (7 7 7 7 7 7 7 7 7 .....)
explode
มันก็คือการสร้าง list ของ (pos x y) char
นั่นเองtest> (explode 0 "hello")
([{:x 0, :y 0} \h] [{:x 1, :y 0} \e] [{:x 2, :y 0} \l] [{:x 3, :y 0} \l] [{:x 4, :y 0} \o])
(reduce into (map ..))
(reduce + [1 2 3 4 5])
=> 15
เขียนอีกอย่างก็คือ
(+ (+ (+ (+ 1 2) 3) 4) 5)
into
นั้นทำงานแบบนี้(into [1] [2])
=> [1 2]
(into [1 2 3] [2])
=> [1 2 3 2]
(into [1] [1 2 3])
=>[1 1 2 3]
test> (line-explode "hello\nworld\n")
([{:x 4, :y 1} \d] [{:x 3, :y 1} \l] [{:x 2, :y 1} \r] [{:x 1, :y 1} \o] [{:x 0, :y 1} \w] [{:x 0, :y 0} \h] [{:x 1, :y 0} \e] [{:x 2, :y 0} \l] [{:x 3, :y 0} \l] [{:x 4, :y 0} \o])